Link to Websites in Scratch Projects with Leopard
So you’re building a Scratch project, and you want to include a link to a website. What can you do?
Scratch doesn’t allow you to open links to websites using code. But that’s okay! There’s a solution.
Convert Your Project to JavaScript with Leopard
Since Scratch can’t open links, we need to convert our project to a different programming language: JavaScript. Fortunately, there’s an easy tool for this.
You can use Leopard to convert your project to JavaScript automatically. Enter your project URL or upload an .sb3 file on the Leopard website:
You’ll be taken to a JavaScript code editor with your project inside. In the left sidebar, you will see folders of code, costumes, and sounds for each of the sprites in your project. Find the sprite you want to edit and open its JavaScript file, which ends in .js.
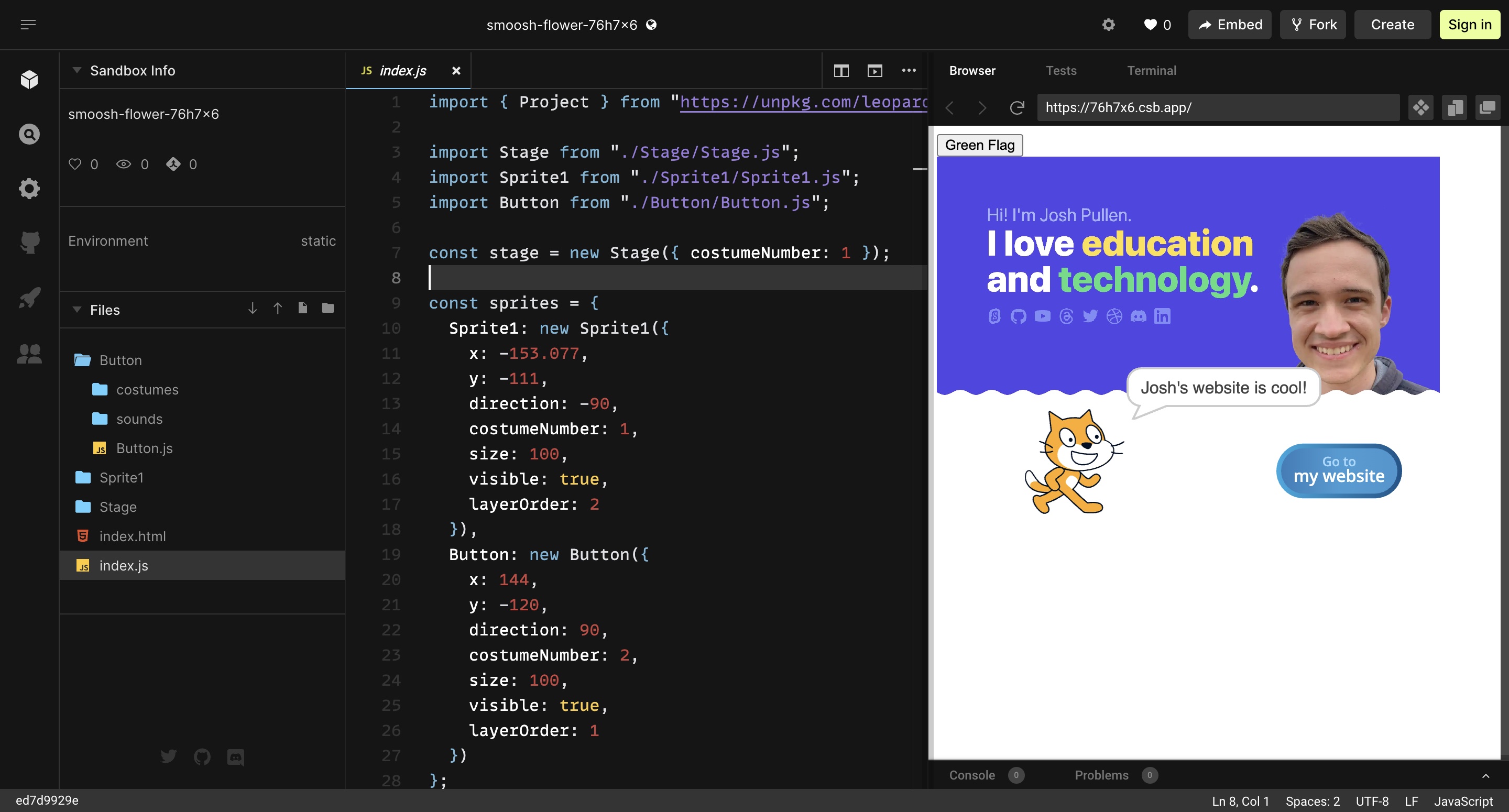
Now we can edit the code! Find the script in your project that you’d like to change. In my case, I want to replace this sayAndWait
block with some code to actually open the website:
// In Button/Button.js
// ...
export default class Button extends Sprite {
constructor(...args) {
// ...
}
*whenthisspriteclicked() {
yield* this.sayAndWait("Go to joshuapullen.com", 2);
}
}
Open a Website Using JavaScript
To open a website using JavaScript, you write the following line:
window.open("https://joshuapullen.com");
Of course, you can change the URL to be any website you want. (You can learn more about the window.open function here.)
In my example, the final code looks like this:
// In Button/Button.js
// ...
export default class Button extends Sprite {
constructor(...args) {
// ...
}
*whenthisspriteclicked() {
window.open("https://joshuapullen.com");
}
}
When the button sprite is clicked, this code will open the webpage in a new tab.
And that’s it! You can now open URLs using JavaScript in your Scratch projects.